The Adapter is a very common Software Design Pattern. Sometimes it is known as Wrapper, often in Projects you'll find Adapters and Wrappers all over.
As in the physical world, for example an USB-Adapter, the Adapter in the software world is very similar. It provides an interface of an existing class to be used by another interface. The Adapter can be implemented either as a Class Adapter Pattern or a Object Adapter Pattern.
Class Adapter Pattern
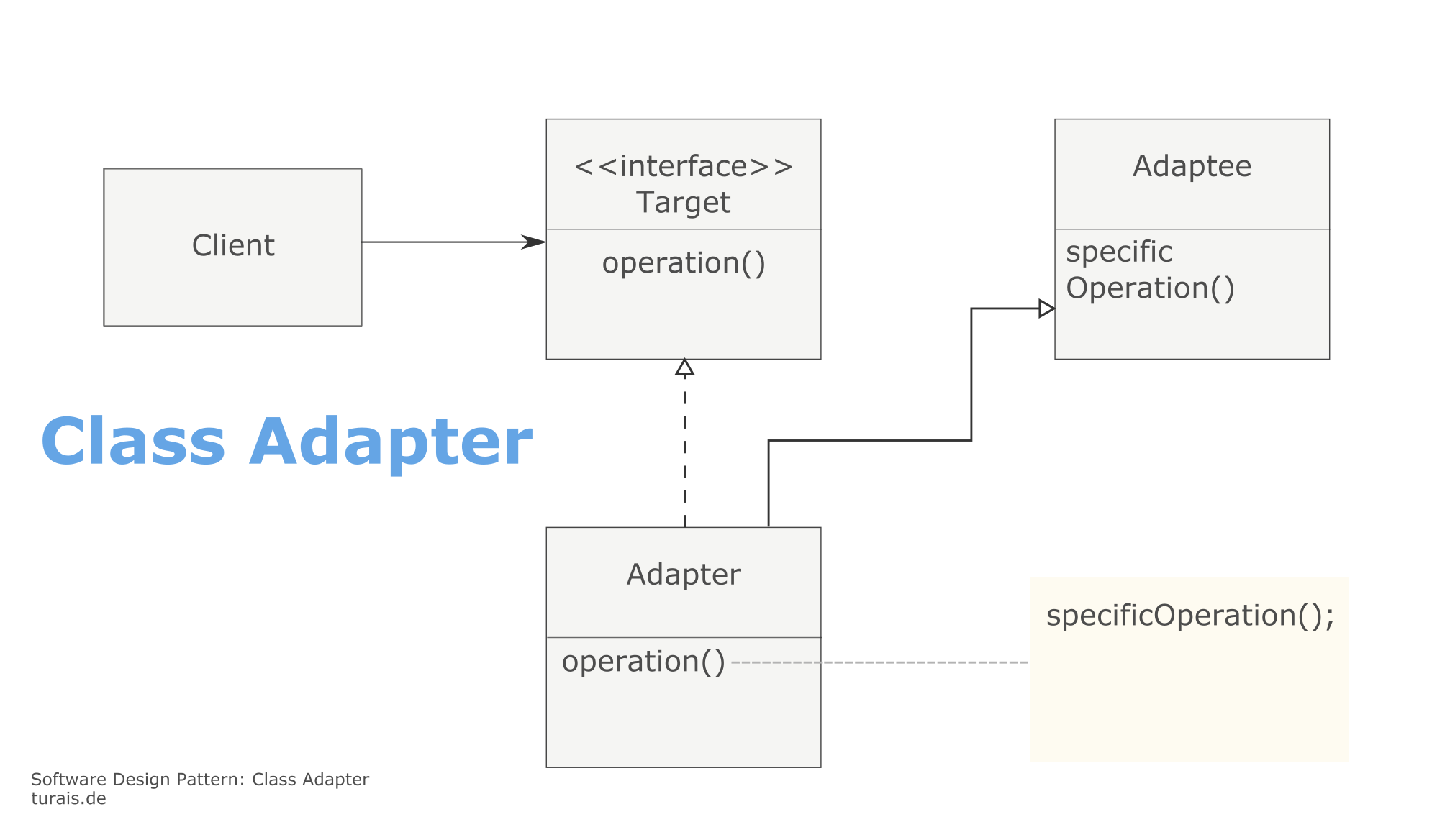
The Class Adapter Pattern is really simple, you build yourself an adapter that "converts" calls to the Adapter to the Adapted. Maybe a working Java example will clarify things:
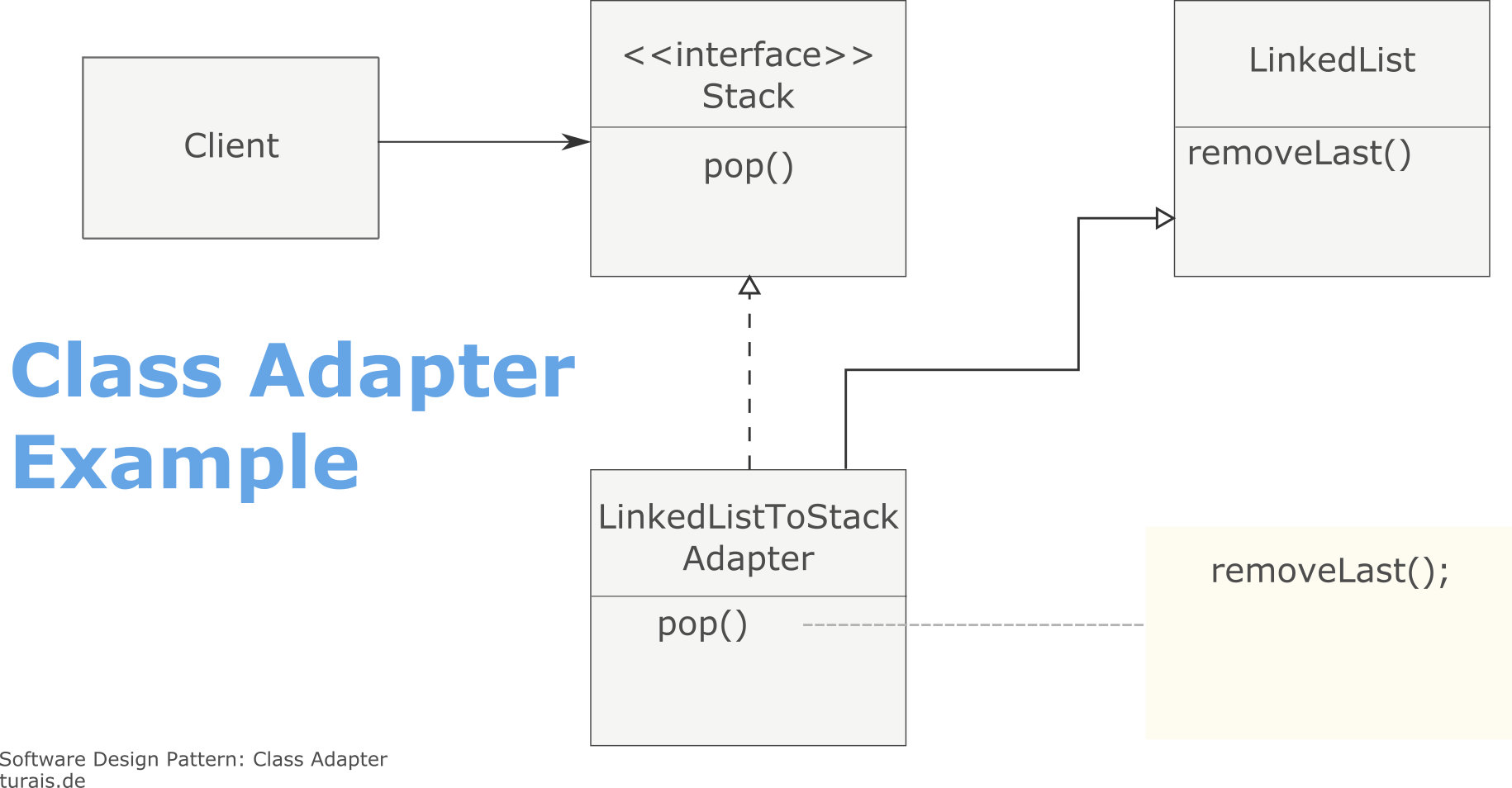
The LinkedList
is a already provided LinkedList that you have (maybe) implemented yourself. Now you want a Stack ability, but when using the Stack the Client should only be able to use Stack-Operations.
This can be done with the Interface Stack shown above. So you'll create:
- The Stack Interface
- The LinkedListToStackAdapter
(Excuse me the CamelCaseWriting ;) )
Your LinkedListToStack would look something like this:
package com.company.adapter;
import java.util.LinkedList;
public class LinkedListToStack extends LinkedList implements Stack {
@Override
public Object pop() {
return super.removeLast();
}
@Override
public void push(Object o) {
super.add(o);
}
}
The overridden-methods are the one from the Stack, we then use specific methods from our super class.
Our Interface Stack should look like this:
package com.company.adapter;
public interface Stack {
public Object pop();
public void push(Object o);
}
And now the Client uses it like this:
package com.company;
import com.company.adapter.LinkedListToStack;
import com.company.adapter.Stack;
public class Main {
public static void main(String[] args) {
Stack stack = new LinkedListToStack();
stack.push(" World!");
stack.push("Hello");
System.out.print(stack.pop());
System.out.println(stack.pop());
}
}
Now the User can use the stack operations like push()
and pop()
.
Object Adapter Pattern
The Object Adapter Pattern differs not much from the Class Adapter Pattern. The Object Adapter Pattern uses Delegation over Inheritance.
What does that mean? Really simple: Instead of using Inheritance, like when you extend a class, it uses an instance (object) of that class. You then use calls to that specific method and wrap that, so that it behaves like your Interface.
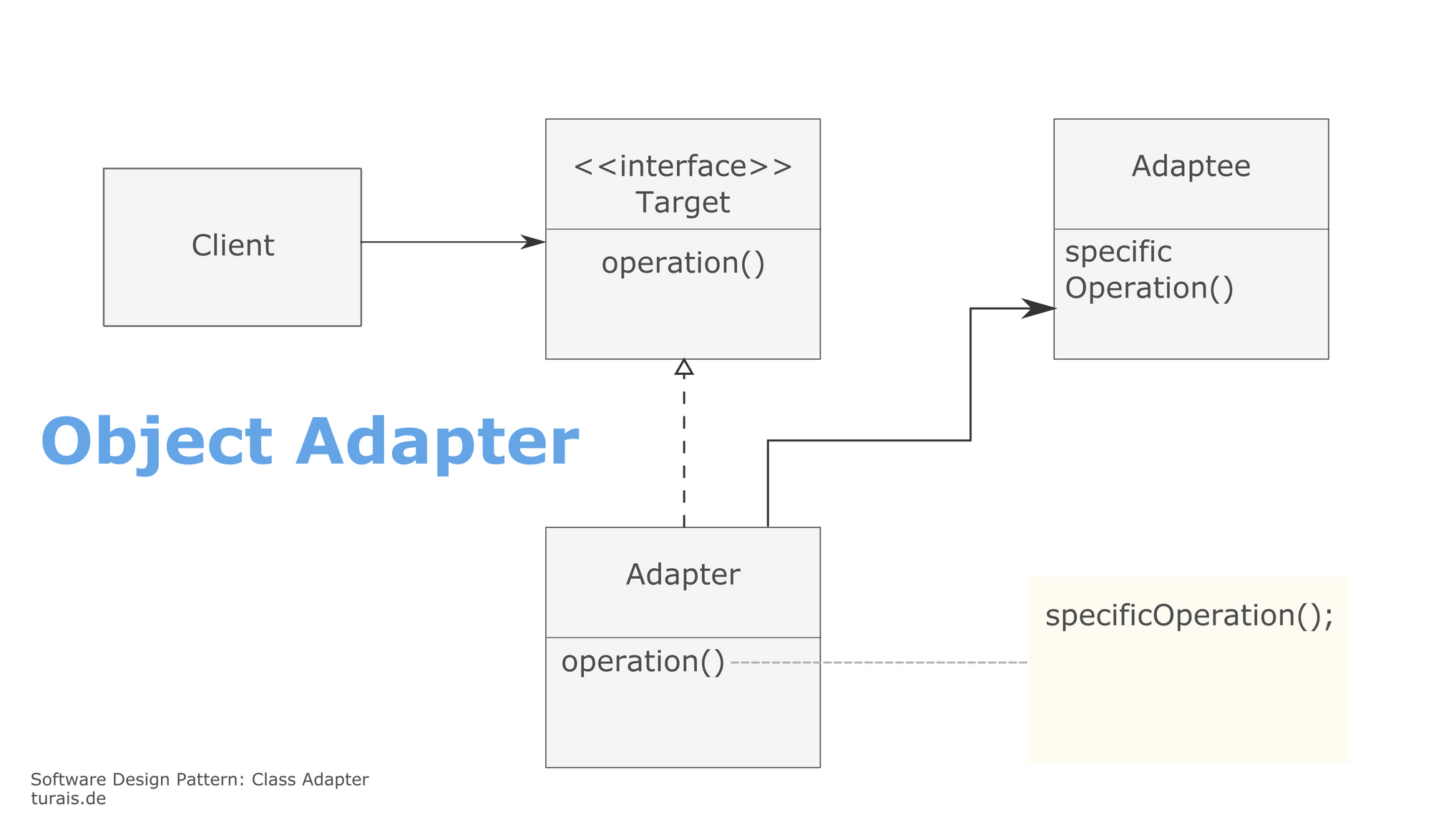
The Adapter now owns an object of Adaptee. You then call member functions of that object to serve the Target's needs.
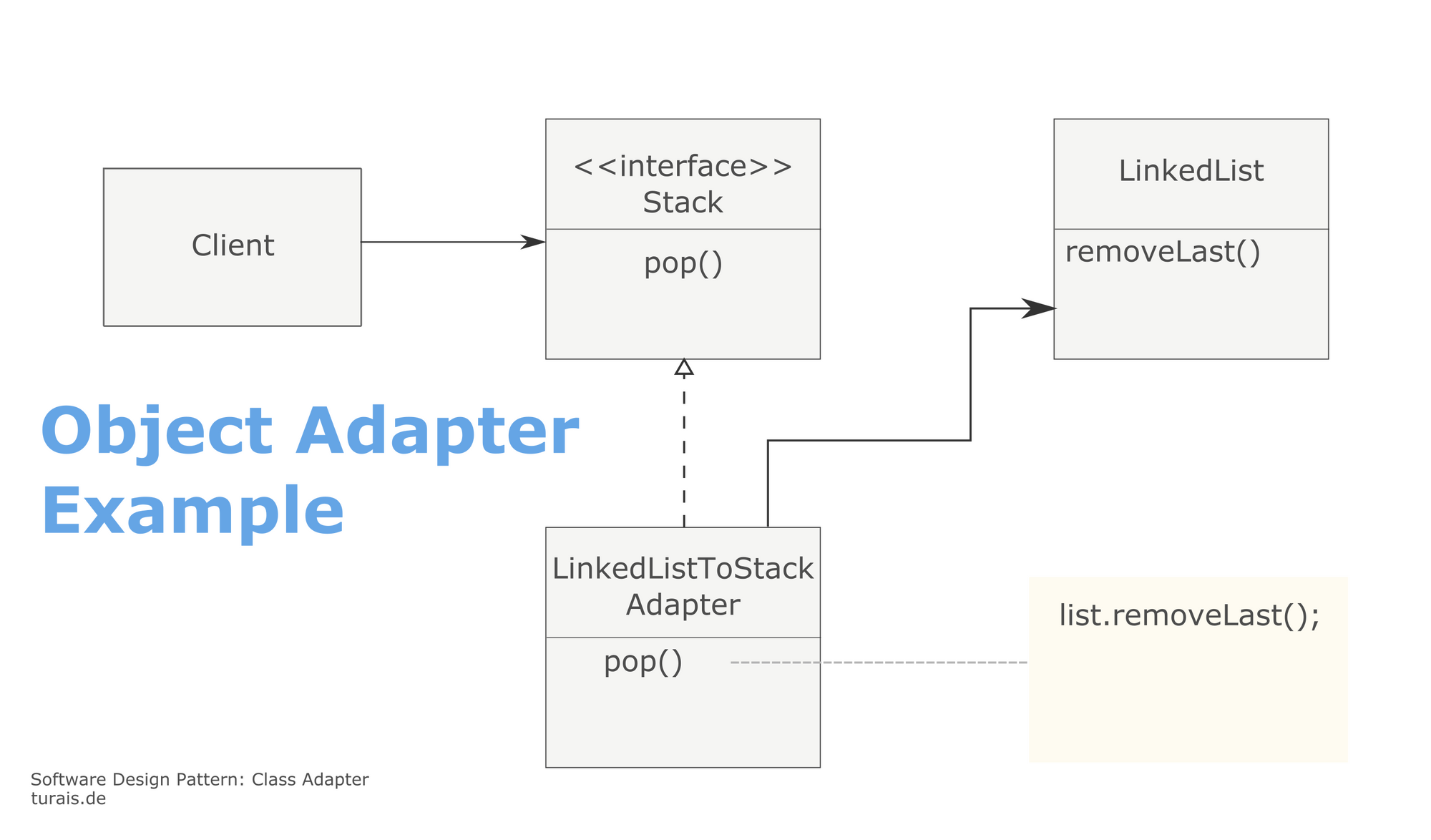
The Example above would be almost identical as the Class Adapter Example. The only thing that changes is the LinkedListToStackAdapter:
package com.company.adapter;
import java.util.LinkedList;
public class LinkedListToStack implements Stack {
private LinkedList<Object> list = new LinkedList<>();
@Override
public Object pop() {
return list.removeLast();
}
@Override
public void push(Object o) {
list.add(o);
}
}
This is part of a mini series if you liked it so far look at other Design Patterns: Click
Book recommendation
Design Patterns - Elements of Reusable Object-Orientated Software
If you press this Button it will Load Disqus-Comments. More on Disqus Privacy: Link